The Popup Scanner
Introduction
Introduced in version 1.6.0 of the SDK, PopupScanner is a pre-built modal scanning dialog that you can integrate into your app with just a single line of code. The entire UI and management of the underlying BarcodeReader is taken care of for you.
It is intended for use cases where tight integration with the host app UX and custom styling is less important.
Example: Scanning a serial number barcode using PopupScanner
Scanning Barcodes
Scanning Single Barcodes
To scan a barcode, call the PopupScanner.scan() method and supply a PopupConfiguration as the argument. The SDK needs to be in an initialized state.
Scanning is an asynchronous operation that returns a Promise, and should be awaited using the await operator. The method returns as soon as a matching barcode is read, and returns undefined
in case the user dismissed the dialog without scanning a barcode.
Example: Scanning Code 128 barcodes using PopupScanner
Scanning Multiple Barcodes
Scanning a sequence of barcodes within the same popup can be achieved by setting a detectionHandler in the Configuration passed to PopupScanner.scan().
The detection handler is a piece of code that indicates to the PopupScanner if scanning should continue or finish after a barcode was detected. Our JavaScript sample on Github illustrates how a detection handler can be used to differentiate between different three different barcodes of the same symbology using regular expressions, and stop scanning once all three have been scanned.
Popup Scanner Elements
The Popup Scanner is a semi-translucent dialog that pops up over your app’s UI. There's a small margin on all sides so it is clear that the popup is shown in the context of your app.
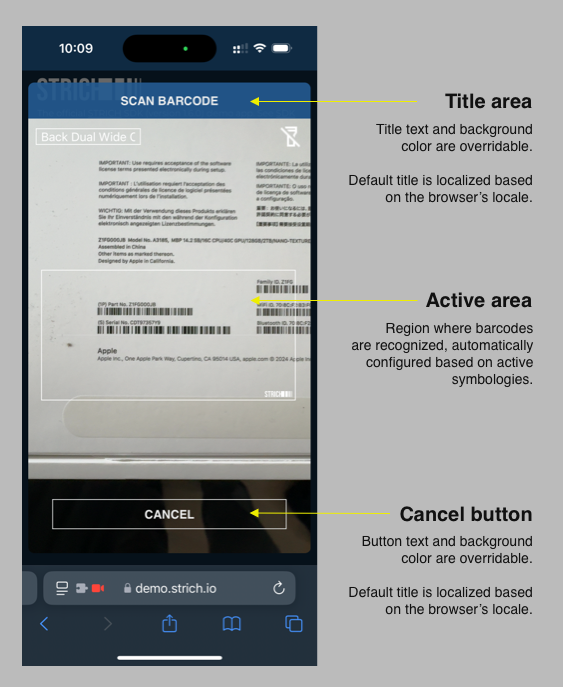
Title Area
The title area is used for user guidance — we recommend using something specific to the use case as the text, like Scan serial number or Scan document ID. The default text is Scan Barcode and is localized based on the browser’s locale. For further customization, a background color matching your app’s color palette can be set.
Active Area
The active area or region of interest is bordered by a rectangle. Its dimensions are set automatically based on the configured 1D and 2D symbologies.
Cancel Button
The cancel button dismisses the dialog, without scanning a barcode. This causes the PopupScanner.scan() to return undefined
. The default text is Cancel and is localized based on the browser’s locale. The text and the button background color can be overridden from their defaults.
Sample Configuration
The following snippet illustrates the full extent of customization that can be achieved through PopupConfiguration.
Sample Code
A vanilla JavaScript sample is available on Github illustrating how to use PopupScanner for filling form inputs.
Requirements
PopupScanner requires browser support for the <dialog> element, a modern Web API which has been widely available since early 2022.